近日,工信部发布《关于推进5G轻量化(RedCap)技术演进和应用创新发展的通知(征求意见稿)》,提出到2025年,5G RedCap产业综合能力显著提升,新产品、新模式不断涌现,融合应用规模上量,安全能力同步增强。其中,全国县级以上城市实现5G RedCap规模覆盖,5G RedCap连接数实现千万级增长。
一时间,RedCap再次成为业界关注的焦点。
那到底什么是RedCap?我们今天来展开讲讲。
蜂窝物联技术演进
先来画一张蜂窝物联技术演进图。
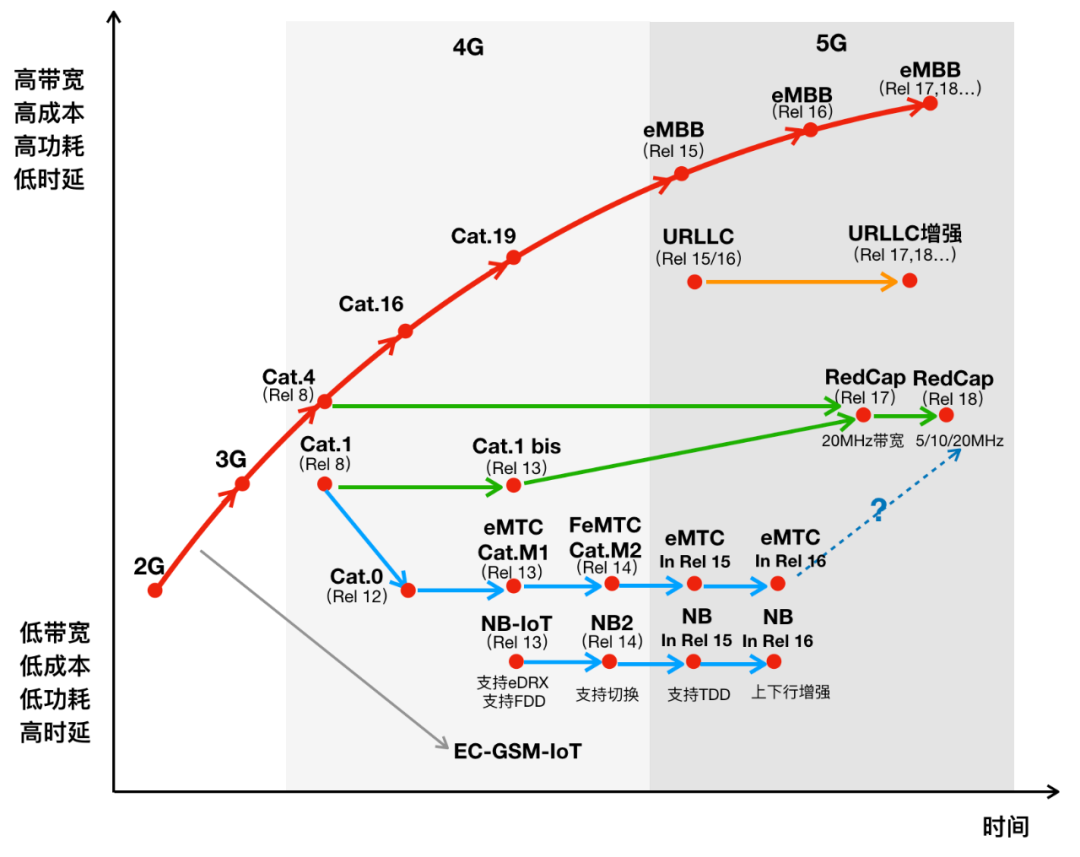
自Release 8以来,在移动宽带(MBB/eMBB)速率不断增长的同时,3GPP也引入了LTE Cat 1、Cat 1 bis、eMTC、NB-IoT、EC-GSM、RedCap等多种蜂窝物联技术。
这些蜂窝物联技术通过不同程度的“功能裁剪”来降低终端和模组的复杂度、成本、尺寸和功耗等指标,从而“量体裁衣”适配不同的物联需求。
简单介绍一下:
LTE Cat 1是3GPP Release 8专门为M2M物联应用制定的蜂窝物联技术,信道带宽为20MHz,下行峰值速率为10Mbps,上行为5Mbps。
LTE Cat1 bis提出于Release 13,作为Cat 1的演进,它进一步将LTE Cat 1终端的双接收天线裁剪为单接收天线。
LTE Cat 0是3GPP Release 12提出的适用于物联应用的UE类型,信道带宽为20MHz,上下行峰值速率为1Mbps。LTE Cat 0支持单接收天线和半双工模式,因此与LTE Cat 1相比,终端复杂度下降至少50%。
在Release 13中,LTE Cat 0演进为eMTC或LTE-M(Cat M1)。由于最大信道带宽缩减至1.4MHz,LTE Cat M1相比LTE Cat 0的复杂度进一步减小,覆盖能力得到进一步提升。
之后,eMTC演进至5G时代的Release 15和Release 16,功能持续增强。
NB-IoT是3GPP Release 13引入的窄带物联技术,信道带宽为180KHz,峰值速率几十至200Kbps左右。
与eMTC一样,NB-IoT也从4G时代的Release 13、Release14持续演进至5G时代,功能不断增强,共同推动5G Massive IoT发展。
EC-GSM-IoT是3GPP Release 13规范的一部分,主要由2G技术升级演变而来,可通过2G GSM网络软件升级实现,信道带宽为200KHz,峰值速率约70至240Kbps。
RedCap制定于5G Release 17版本,全称Reduced Capability NR,即“裁剪NR能力”的意思,所以也被称为轻量版、简化版的5G NR。
为什么要引入RedCap?
众所周知,5G最初定义了eMBB、uRLLC和mMTC三大场景,与之相对应,5G时代的物联网类型包括宽带物联网、关键任务型物联网和大规模物联网。
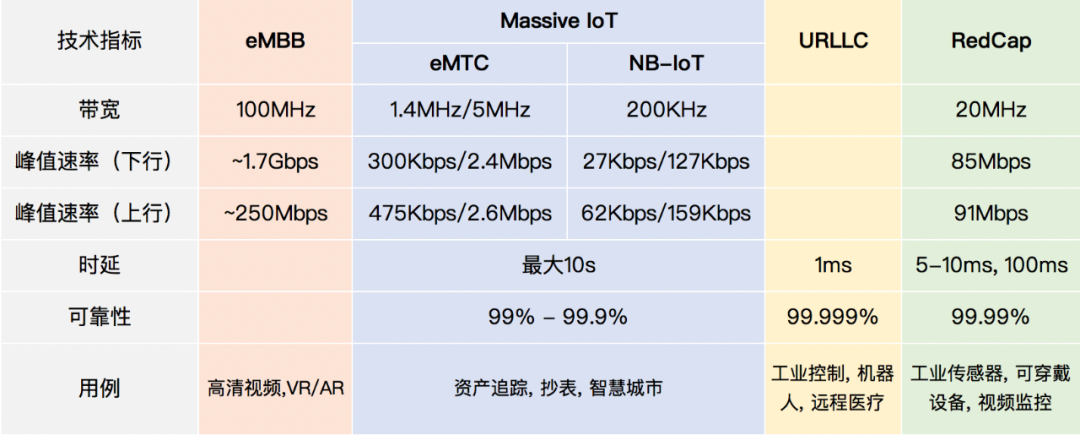
eMBB主要针对高清视频、VR/AR等大带宽应用,通常网络下行峰值速率高达Gbps,上行达几百Mbps。
uRLLC主要针对远程控制、无人驾驶等关键任务型物联网应用,要求网络端到端时延低至毫秒级,可靠性高达5个9。
而mMTC由4G时代的NB-IoT和eMTC低功耗广域物联网技术演进而来,主要面向智能抄表、智能路灯等大规模物联网场景,对数据传输速率和时延要求很低,网速小于2Mbps,时延可容忍至10秒。
虽然这三大场景差异化匹配了高速率、低时延和低速率的物联应用需求,但细心的你一定发现了,那些对速率和时延要求中等的物联网应用还没有覆盖到。
比如,用于测量和采集温度、湿度、压力等数据的工业无线传感器要求数据传输速率约2Mbps,时延小于100毫秒,可靠性仅需99.99%;智能手表、医疗监控设备、AR/VR眼镜等可穿戴设备要求数据传输速率约2至10Mbps;监控摄像头要求数据传输速率约2至25Mbps,时延小于500毫秒,可靠性仅需99%至99.9%。
这些物联网场景如果用eMBB和uRLLC能力来支撑,就是大材小用、资源浪费,而mMTC的速率和时延能力又不能满足需求。
RedCap,正是为了填补eMBB、mMTC与uRLLC之间的“空白地带”而生的蜂窝物联技术。
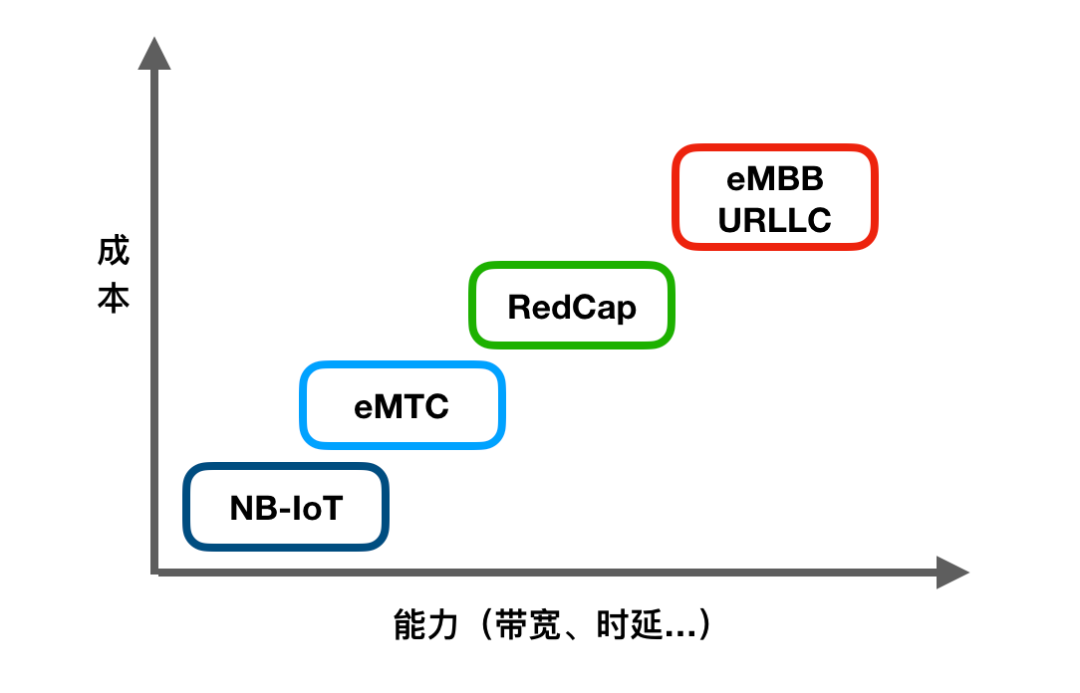
正如演进图中所示,RedCap位于中间位置。如果根据带宽、时延、成本、终端功耗、覆盖距离等技术指标将5G蜂窝物联网分为低、中、高三个档位,那么RedCap就是5G时代的“中档”物联技术。
RedCap裁剪了哪些能力?
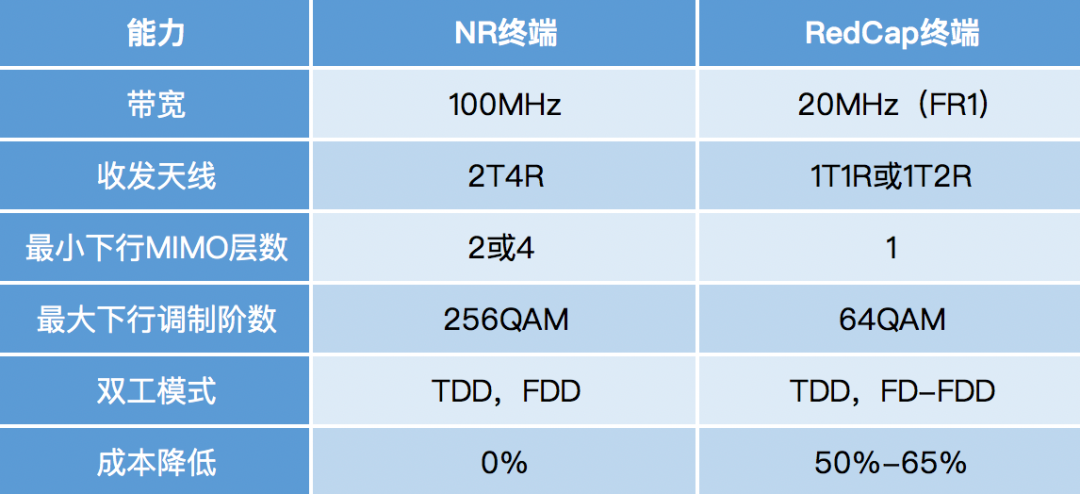
640?wx_fmt=png
如上表,Release 17 RedCap主要裁剪了以下功能,从而将终端复杂度和成本下降50%至65%。
- 最大带宽从NR的100MHz缩减为20MHz
- 天线配置从NR的2T4R减少到1T1R或1T2R
- 最小下行MIMO层数减少至1
- 最大调制阶数最低可支持64QAM
- 引入了半双工模式
为了实现更高的峰值速率,RedCap终端设备也可以不用裁剪得这么狠,可以选择更高级的功能,比如支持2个接收天线、2个下行MIMO层、256QAM、全双工FDD等。
通过这些能力剪裁,可更加低成本、高效率地匹配可穿戴设备(包括可穿戴手表、AR/VR眼镜等)、工业无线传感器、监控摄像头等物联应用的网络连接需求。
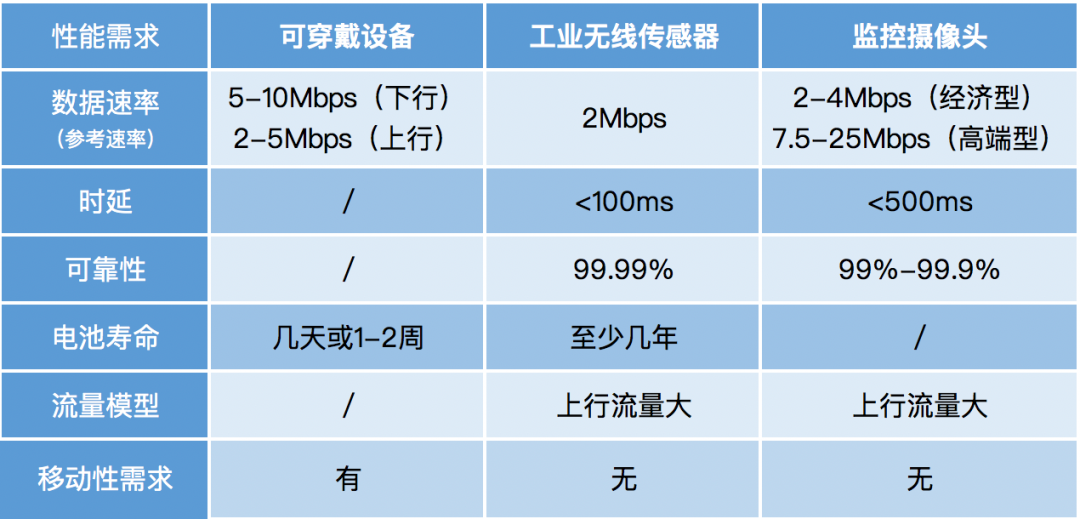
同时,针对可穿戴设备、工业无线传感器等通常没有外部供电条件的场景,要求电池使用寿命长达1-2周甚至数年,RedCap还通过降低终端发射功率等级、引入eDRX和RRM测量放松等节电技术,来进一步降低终端功耗。
RedCap将取代谁?
比较一下技术指标,很明显,RedCap主要将取代4G时代的LTE Cat 4和LTE Cat 1/1 bis。
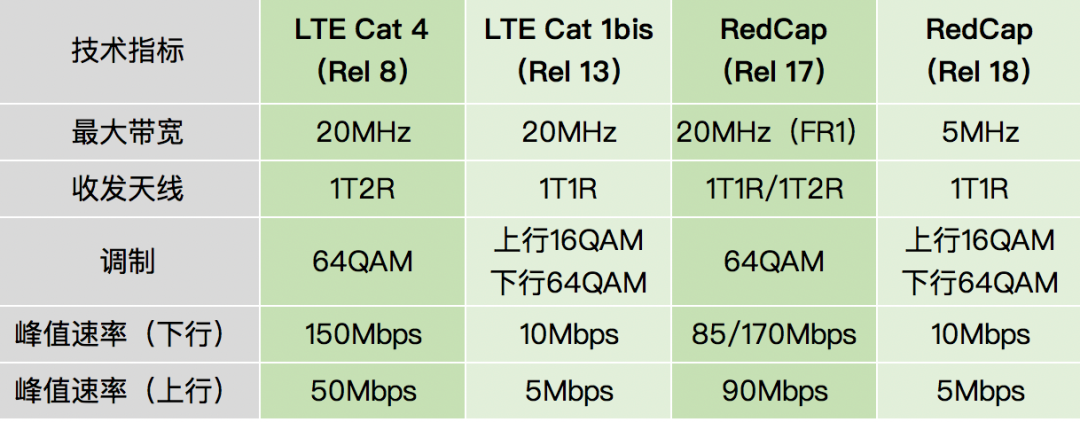
准确点讲,Release 17版本的RedCap预计将取代基于LTE Cat 4的物联应用。而Release 18版本中的RedCap,即增强版RedCap预计将取代基于LTE Cat 1和LTE Cat 1 bis的物联应用。
在R18版本中,增强版的RedCap或将增加支持5MHz、10MHz和40MHz带宽,并进一步降低终端复杂度和成本,以及增加定位、sidelink连接等功能,从而支撑更广泛的物联应用。
为啥要取代LTE Cat 4和Cat 1/1bis?
首先,RedCap为4G时代的LTE Cat1/1 bis和Cat 4提供了向5G网络迁移的路径,面向未来利于运营商重耕4G LTE频谱资源。据统计,当前LTE Cat 1 bis 和 Cat 4 约占当前全球蜂窝物联网市场的一半。
其次,与LTE Cat 4和Cat 1/1bis相比, RedCap天然具有5G能力优势,比如,不仅支持包括毫米波在内的更广泛的频段、网络效率更高、具备5G安全能力,而且可与5G网络切片、5G专网、5G定位等技术结合提供更好的物联服务,同时理论上运营商只需对现有5G网络进行软件升级即可部署。
另外,RedCap填补了中档物联空白,使5G具备了一张网络适配所有低、中、高档物联应用的能力,这也利于蜂窝物联网统一高效管理。
转载自:https://www.txrjy.com/thread-1297962-1-1.html
Views: 12